티스토리 뷰
MVC (Model + View + Controller)
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/tictactoe"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingTop="44dp"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:gravity="center_horizontal"
tools:context=".MainActivity">
<GridLayout
android:id="@+id/buttonGrid"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:rowCount="3"
android:columnCount="3">
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="00"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="01"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="02"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="10"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="11"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="12"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="20"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="21"
android:onClick="onCellClicked" />
<Button
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="30sp"
android:tag="22"
android:onClick="onCellClicked" />
</GridLayout>
<LinearLayout
android:id="@+id/winnerPlayerViewGroup"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical"
android:visibility="gone"
tools:visibility="visible">
<TextView
android:id="@+id/winnerPlayerLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="40sp"
android:layout_margin="20dp"
tools:text="X" />
<TextView
android:id="@+id/asdad"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="Winner" />
</LinearLayout>
<Button
android:id="@+id/btnReset"
android:layout_width="200dp"
android:layout_height="70dp"
android:layout_marginTop="40dp"
android:textAllCaps="false"
android:text="Reset"
android:textSize="20dp" />
</LinearLayout>
View 역할
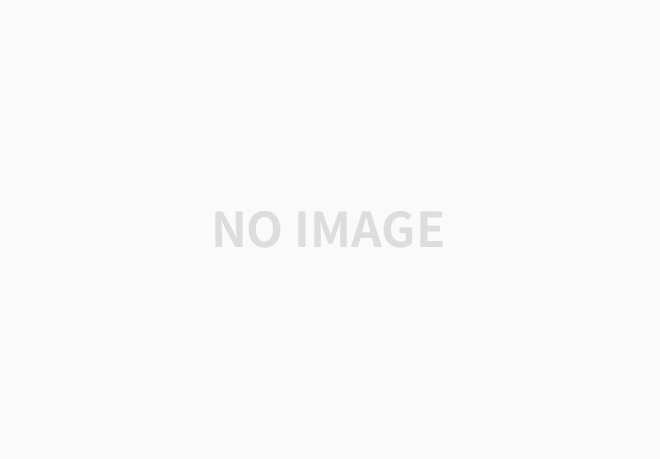
model/Player.java
package com.jwsoft.model;
public enum Player {
O,
X
}
model/Cell.java
package com.jwsoft.model;
public class Cell {
private Player value;
public void setValue(Player value) {
this.value = value;
}
public Player getValue() {
return value;
}
}
model/Board.java
package com.jwsoft.model;
public class Board {
private Cell[][] cells = new Cell[3][3];
private Player winner; // 승리한 사람
private Player currentTurn; // 현재 차례 플레이어
private GameState state; // 게임 진행 상태
private enum GameState { IN_PROGRESS, FINISHED }
public Board() {
restart();
}
public void restart() {
clearCells(); // 모든 셀 초기화
winner = null;
currentTurn = Player.X; // X부터 시작
state = GameState.IN_PROGRESS;
}
private void clearCells() { // 모든 셀 초기화
for(int i=0; i<3; i++) {
for (int j=0; j<3; j++) {
cells[i][j] = new Cell();
}
}
}
public Player mark( int row, int col ) {
Player playerThatMoved = null; // 움직인 선수 초기화
if(isValid(row, col)) { // 해당 셀을 선택할 수 있으면
cells[row][col].setValue(currentTurn); // 해당 셀에 현재 차례의 선수 삽입
playerThatMoved = currentTurn; // 현재 차례 선수를 움직인 선수에 삽입
if(isWinningMoveByPlayer(currentTurn, row, col)) { // 승부가 났는지 확인
state = GameState.FINISHED;
winner = currentTurn;
} else {
// flip the current turn and continue
flipCurrentTurn(); // 현재 차례 선수 바꿈.
}
}
return playerThatMoved;
}
public Player getWinner() {
return winner;
}
private boolean isValid(int row, int col ) { // 해당 셀이 선택 가능한지 여부
if( state == GameState.FINISHED ) {
return false;
} else if( isOutOfBounds(row) || isOutOfBounds(col) ) {
return false;
} else if( isCellValueAlreadySet(row, col) ) { // 이미 선택된 셀이면
return false;
} else {
return true;
}
}
private boolean isOutOfBounds(int idx) {
return idx < 0 || idx > 2;
}
private boolean isCellValueAlreadySet(int row, int col) {
return cells[row][col].getValue() != null;
}
private boolean isWinningMoveByPlayer(Player player, int currentRow, int currentCol) {
return (cells[currentRow][0].getValue() == player // 3-in-the-row
&& cells[currentRow][1].getValue() == player
&& cells[currentRow][2].getValue() == player
|| cells[0][currentCol].getValue() == player // 3-in-the-column
&& cells[1][currentCol].getValue() == player
&& cells[2][currentCol].getValue() == player
|| currentRow == currentCol // 3-in-the-diagonal
&& cells[0][0].getValue() == player
&& cells[1][1].getValue() == player
&& cells[2][2].getValue() == player
|| currentRow + currentCol == 2 // 3-in-the-opposite-diagonal
&& cells[0][2].getValue() == player
&& cells[1][1].getValue() == player
&& cells[2][0].getValue() == player);
}
private void flipCurrentTurn() {
currentTurn = currentTurn == Player.X ? Player.O : Player.X;
}
}
Model 역할
MainActivity.java
package com.jwsoft.javaproject;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
import com.jwsoft.model.Board;
import com.jwsoft.model.Player;
// Controller 역할.
public class MainActivity extends AppCompatActivity {
private static String TAG = MainActivity.class.getName();
private Board model; // 모델 객체 생성 (Model)
private ViewGroup buttonGrid; // 뷰 객체 생성 (View)
private View winnerPlayerViewGroup; // 뷰 객체 생성 (View)
private TextView winnerPlayerLabel; // 뷰 객체 생성 (View)
private Button btnReset; // 뷰 객체 생성 (View)
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
winnerPlayerLabel = findViewById(R.id.winnerPlayerLabel); // 뷰 객체 생성 (View)
winnerPlayerViewGroup = findViewById(R.id.winnerPlayerViewGroup); // 뷰 객체 생성 (View)
buttonGrid = findViewById(R.id.buttonGrid); // 뷰 객체 생성 (View)
btnReset = findViewById(R.id.btnReset); // 뷰 객체 생성 (View)
btnReset.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
reset(); // 사용자가 View 클릭하면 Controller에서 작업 처리
}
});
model = new Board();
}
public void onCellClicked(View v) {
Button button = (Button) v;
String tag = button.getTag().toString();
int row = Integer.parseInt(tag.substring(0, 1));
int col = Integer.parseInt(tag.substring(1, 2));
Log.i(TAG, "Click Row : [" + row + ", " + col + "]");
Player playerThatMoved = model.mark(row, col);
if (playerThatMoved != null) {
button.setText(playerThatMoved.toString()); // View와 Model의 의존성, 결합도 증가.
if (model.getWinner() != null) { // View와 Model의 의존성, 결합도 증가.
winnerPlayerLabel.setText(playerThatMoved.toString());
winnerPlayerViewGroup.setVisibility(View.VISIBLE);
}
}
}
private void reset() { // Controller가 작업 처리
winnerPlayerViewGroup.setVisibility(View.GONE); // Controller -> View
winnerPlayerLabel.setText(""); // Controller -> View
model.restart(); // Controller -> Model
for (int i=0; i < buttonGrid.getChildCount(); i++) {
((Button) buttonGrid.getChildAt(i)).setText("");
}
}
}
Controller 역할
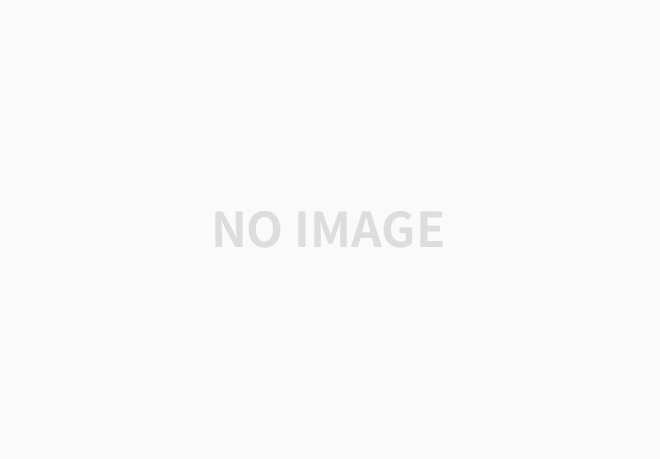
참고.
academy.realm.io/kr/posts/eric-maxwell-mvc-mvp-and-mvvm-on-android/
안드로이드의 MVC, MVP, MVVM 종합 안내서
안드로이드 앱을 만드는 개발자를 위한 MVC, MVP, MVVM 패턴 사용법과 장단점에 대한 안내서입니다.
academy.realm.io
github.com/ericmaxwell2003/ticTacToe
ericmaxwell2003/ticTacToe
A simple tic tac toe app, to illustrate the use of MVC, MVP, and MVVM architectures to organize the application. - ericmaxwell2003/ticTacToe
github.com
'Android > Java' 카테고리의 다른 글
[Java] Tic-tac-toe 게임으로 보는 MVVM 패턴 (0) | 2020.09.19 |
---|---|
[Java] Tic-tac-toe 게임으로 보는 MVP 패턴 (0) | 2020.09.18 |
[Java] Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_CLEAR_TASK (0) | 2020.09.02 |
[Java] InputMethodManager (0) | 2020.08.25 |
[Java] STT (Speech-to-Text) (0) | 2020.08.24 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- handler
- ViewModel
- activity
- TabLayout
- Android
- Kotlin
- Design Pattern
- JSONArray
- ArrayList
- Vue.js #Vue.js + javascript
- DataBinding
- coroutine
- 혀가 길지 않은 개발자
- 안드로이드
- ViewPager2
- MVVM
- Livedata
- James Kim
- 자바
- 안드로이드 #코틀린 #Android #Kotlin
- XML
- fragment
- CoordinatorLayout
- Intent
- java
- 코틀린
- Architecture Pattern
- View
- JSONObject
- recyclerview
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
글 보관함